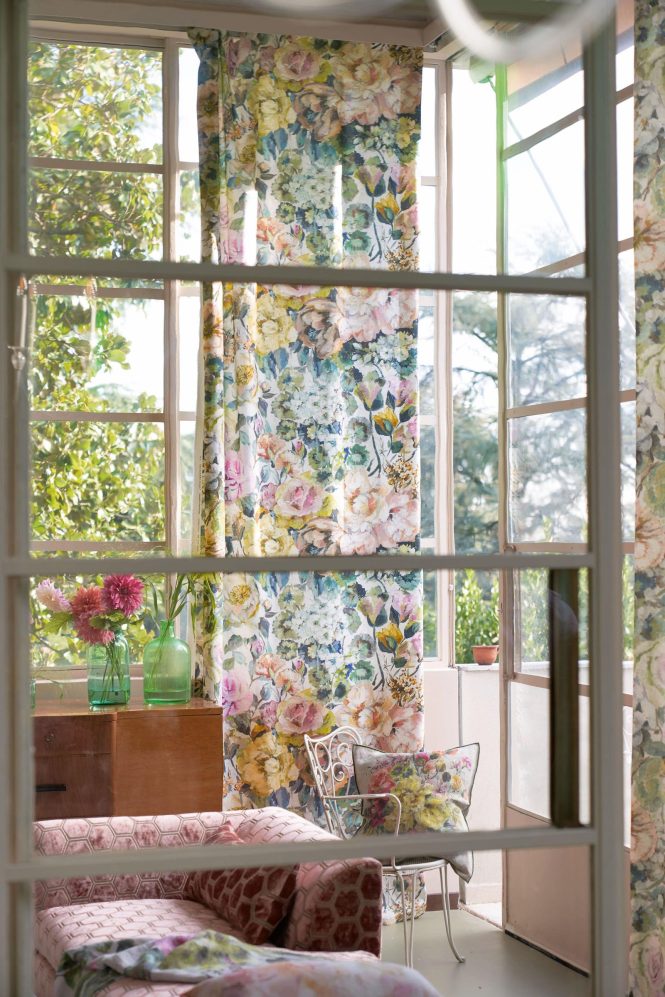
Solving Decorator Pattern Clashes is a critical aspect of software development, particularly in object-oriented programming. The Decorator pattern offers a flexible way to enhance objects dynamically, but clashes can arise from complex interdependencies. This guide explores strategies to effectively troubleshoot these problems, ensuring code maintainability and scalability. Common issues include compatibility problems between decorators, unforeseen side effects from chained decorators, and conflicts in the pattern’s implementation. This article examines these problems thoroughly and provides practical solutions with examples to illustrate the concepts. We’ll cover the various approaches to diagnosis and fix, focusing on how to identify, understand, and resolve conflicts with the decorator pattern. This guide is structured into sections that will help you master the process of troubleshooting decorator clashes.
Understanding the Decorator Pattern
Core Concepts of the Decorator Pattern
The Decorator pattern is a powerful design pattern that allows you to dynamically add functionalities to an object without modifying its class. It works by wrapping the original object with decorator objects, each adding specific behavior. This approach promotes flexibility and allows for reusable components. Understanding this core concept is crucial for troubleshooting potential conflicts. Consider the case of graphical user interface (GUI) elements, where the addition of borders, shadows, or special effects can be handled easily through decorators. This modularity makes the Decorator Pattern a popular choice in systems needing to adapt dynamically.
Common Issues With the Decorator Pattern
However, the very flexibility inherent in the Decorator Pattern can sometimes lead to complex interdependencies and conflicts. Unexpected interactions between decorators may alter the expected behavior of the original object. Compatibility issues arise when decorators don’t function correctly when chained together. Poorly designed decorators can lead to clashes during runtime.
Identifying the Source of Conflicts
Thorough testing and understanding the inner workings of the decorator chain are essential to pinpoint the source of issues. Method tracing through the chain of decorators is vital. Examining the method call flow helps in locating points of clash or unexpected behavior. A meticulous approach to debugging helps determine which decorator causes the incompatibility.
Diagnosing Decorator Conflicts
Analyzing Dependencies
Thorough analysis of dependencies among decorators is a crucial step in isolating conflicts. Visualize the decorator chain and method calls. This detailed map will help you pinpoint potential issues. Look for conflicts where decorators modify or interact with each other’s internal states or methods. This analysis will identify unexpected interactions.
Identifying Conflicts in the Decorator Chain
One way to troubleshoot is by identifying the exact method or step where the conflict originates. Understanding the specific method calls involved provides valuable clues to the source of the conflict. Use logging or debugging tools to track the flow of execution and highlight the moments of incompatibility.
Debugging Techniques and Best Practices
Modern IDEs provide robust debugging tools to isolate the exact decorator responsible. Using these tools helps step through the execution flow, monitoring the behavior of each decorator. This detailed analysis helps to pin down the issues.
Resolving Decorator Conflicts
Refactoring Techniques
Refactoring is crucial for resolving Decorator clashes. Identify and resolve the root cause of the problem in the decorator code by examining the interactions between the decorators. Extract or refactor existing code into new modules if necessary. Refactoring existing code can make your codebase less prone to errors. For example, introducing a new decorator that encapsulates the common functionality that causes issues or breaking down a large decorator into smaller, more focused ones can help simplify and improve the overall efficiency of the Decorator chain.
Isolation and Testing
Isolate the faulty decorator and its interactions through targeted testing. Writing unit tests focused on specific methods or parts of the decorator chain helps you understand interactions. Isolate the code sections causing the conflict. By writing comprehensive test cases focused on specific methods or aspects of the decorates chain, we can ensure compatibility and fix conflicts early on. This isolation process allows for a focused evaluation of potential conflicts and enables targeted modifications.
Comprehensive Testing Strategy
Develop a testing strategy to prevent similar conflicts. Comprehensive testing helps to reveal potential conflicts and ensure that all decorators work in the anticipated manner. Create a robust test environment to cover various input conditions. This enables the identification and resolution of conflicts early in the development lifecycle.
Case Study: GUI Decorator Conflicts
Scenario
Imagine a graphical user interface (GUI) where buttons can have multiple visual enhancements: a border, a shadow, and a highlight. Each feature is represented by a decorator. In one specific scenario, the chain of decorators caused a color overflow in the button appearance. This specific example highlights that the decorator chain might introduce side effects, which requires careful design and testing to prevent.
Analysis and Resolution
Upon investigation, it was found that the color calculation in the highlight decorator interfered with the border color. This suggests that the calculation for highlighting affected the final color result of the button. The solution was to refactor the color calculation in the highlighting decorator to avoid overwriting values in the original color.
Prevention Strategies
By adding input validation in the decorators and careful handling of method calls, such conflicts can be avoided. Adding thorough testing procedures to the development workflow would allow the identification of these type of conflicts. Ensuring that each decorator operates independently and with minimal interference will help prevent future conflicts.
Best Practices for Decorator Design
Clear Interface Definition
A clear and concise interface specification is vital to prevent ambiguity and maintain consistency. The decorator pattern is most beneficial when interfaces are well-defined and understood throughout the software design. Defining clear methods reduces possible conflicts.
Modular Design
Design decorators as independent, reusable units. Keep them focused on a specific aspect. Dividing decorators into separate modules enhances modularity and maintainability. This modularity makes the Decorator Pattern a popular choice in systems needing to adapt dynamically.
Thorough Testing and Validation
Adopt a comprehensive testing strategy. Create unit tests to cover all aspects of decorator interactions, and simulate realistic use cases. Testing is crucial to identifying conflicts early on in the design cycle.
Specific scenarios for decorator conflicts
Examples of resolving decorators
Frequently Asked Questions
What are the common causes of conflicts in the Decorator Pattern?
One of the common causes of decorator pattern conflicts is improper method call interactions and unexpected behavior from multiple decorators interacting. Incorrect ordering or interactions between decorators can lead to unexpected modifications or conflicts. Lack of testing for these situations results in runtime errors and conflicts that are harder to resolve at later stages. Another common source is incorrect handling of input parameters or states, leading to unintended consequences and possible conflicts when multiple decorators modify the same property or attribute of the target object. Understanding these root causes allows for the implementation of preventative measures.
How do I identify the source of a Decorator Pattern conflict?
To identify the source of the decorator pattern conflict, start with method tracing. Tracking the order in which methods are called through the chain of decorators can pinpoint the specific point of incompatibility. Use logging or debugging tools to inspect intermediate states and identify the specific decorator that introduces the undesirable behavior. Visualizing the object-decorator chain and their relationships is also an effective strategy. Method call sequences and interaction diagrams can help uncover specific points of conflict and identify the specific decorator responsible.
In conclusion, resolving Decorator Pattern clashes requires a meticulous understanding of the pattern’s implementation and potential conflicts. By carefully analyzing dependencies, identifying the source of conflicts, and employing refactoring techniques, developers can effectively address these challenges. This guide provides practical steps for troubleshooting Decorator Pattern conflicts. By implementing these strategies, software systems can maintain consistency, improve readability, and ensure scalability. To delve deeper into this topic, explore further resources on design patterns and software architecture. Start using these strategies today!